Salesforce Apex Trigger to Prevent the user from creating Duplicate Contacts when a Contact already exists with the same Email or Phone number – Triggers for Beginners
The requirement is:
When a Contact is being created, if a record with the same Email or Phone number exists, the trigger should throw an error and the user should not be allowed to create the duplicate Contact.
To achieve this, we will write a trigger with the following flow:
- Define the Trigger
- Create Sets to store the ‘Email Ids’ and ‘Phone Numbers’ of all the records that are being created
- Iterate through each record that is being created, and add their ‘Email Ids’ and ‘Phone Numbers’ to the Sets.
- Create a List to store the ‘Email Ids’ and ‘Phone Numbers’ of existing records that match the records being created.
- Populate the above List with of existing records whose email id or phone number matches the ones being created.
- Iterate through each record being created and display and error if the record already exists.
Now lets go through each step once again and write its respective code.
The complete trigger to prevent duplicate contacts by matching email or phone number and displaying the error on the email field would be:
trigger PreventDuplicateContacts on Contact (before insert) { // Set to store email ids Set <String> emailSet = new Set<String>(); // Set to store phone numbers Set <String> phoneSet = new Set<String>(); // Iterate through each Contact and add their email and phone number to their respective Sets for (contact con:trigger.new) { emailSet.add(con.email); phoneSet.add(con.phone); } // New list to store the found email or phone numbers List <Contact> contactList = new List<Contact>(); // Populating the list using SOQL contactlist = [SELECT email,phone FROM Contact WHERE email IN :emailSet OR phone IN :phoneSet]; // Iterating through each Contact record to see if the same email or phone was found for (contact con:trigger.new) { If (contactList.size() > 0) { // Displaying the error con.email.adderror( 'Duplicate Contact Found. Use Existing Contact.' ); } } }
Here is how the Trigger to Prevent Duplicate Contacts looks in the Salesforce Developer Console (image)
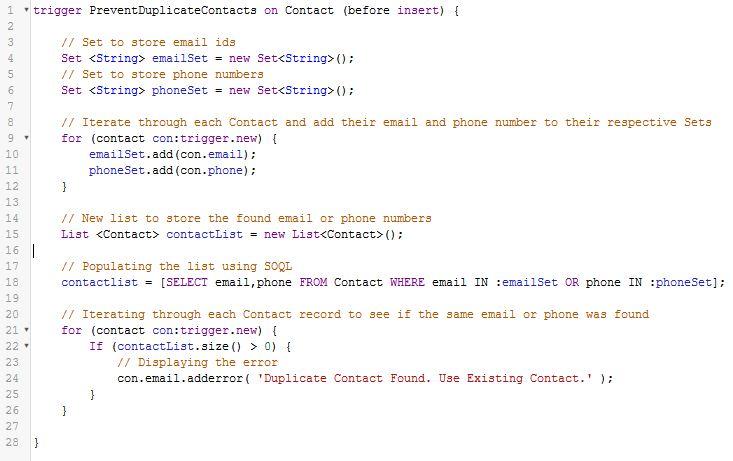
Leave a Reply